[Swift]AlamofireとKannaでスクレイピングをする
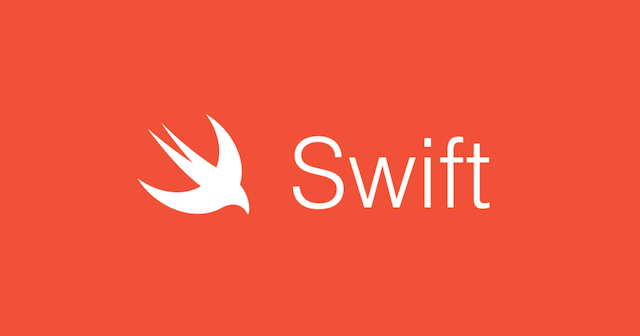
SwiftでWebサイトをスクレイピングしてみます。
AlamofireとKannaを使います。
やること
かなり簡単なサンプルを作ってみます。あるサイトにアクセスして、サクッとクローリングします。
使うのは、AlamofireとKannaというライブラリ。
さっそく見ていきましょう。
Xcodeでプロジェクトを作る
まずは、いつものようにXcodeでプロジェクトを立ち上げます。プロジェクト名は適当に。
次に、CocoapodsからAlamofireとKannaをインストールします。
Alamofireというのは、Webサイトにリクエストを送るやつでKannaは取得したhtmlコードをパースするやつです。
iOSアプリ開発でCocoapodsが使えるように環境を作る方法
cocoapodsをインストールする。エラーに会った時やインストール後の対応まとめ
cocoapodsをインストールしていきます。ターミナルを立ち上げて、プロジェクトまで移動します。
xxx-no-MacBook-Pro:testClawler xxx$ pod init
移動したところで、pod initでPodfileを作成します。
次にopen Podfileでファイルを開きます。(Finderから開いてもOK)
xxx-no-MacBook-Pro:testClawler xxx$ open Podfile
Podfileには以下のように記述します。
# Uncomment the next line to define a global platform for your project # platform :ios, '9.0' target 'testClawler' do # Comment the next line if you're not using Swift and don't want to use dynamic frameworks use_frameworks! # Pods for testClawler # 以下2つを追記 pod "Alamofire" pod "Kanna" end
余談ですけど、PodfileってRubyで書かれているんですよね。
podを追記し保存したところで、ターミナルに戻りpod installします。
xxx-no-MacBook-Pro:testClawler xxx$ pod install
これで、プロジェクトにAlamofireとKannaを反映させる準備ができました。ここでいったんXcodeを閉じて、再度白色のファイルを開くようにします。
ファイルを開いて、importしておきます。
import UIKit import Alamofire import Kanna class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad()
これで、下準備はOKです。次で実際にサイトにリクエストを送る→パースするコードを書いていきます。
コードを書く
コード全文を載せます。
import UIKit import Alamofire import Kanna class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() scrapeWebsite() } func scrapeWebsite() { //GETリクエスト 指定URLのコードを取得 Alamofire.request("https://www.yahoo.co.jp").responseString { response in print("\(response.result.isSuccess)") if let html = response.result.value { self.parseHTML(html: html) } } } func parseHTML(html: String) { if let doc = try? HTML(html: html, encoding: .utf8) { print(doc.title) for link in doc.css("a, link") { print(link.text) print(link["href"]) } } } }
今回はtitleタグとリンクタグを取得しました。
Alamofireで指定URLにGETリクエストを送って、Kannaでパースします。
詳しい使い方はGithubを見てください。
https://github.com/Alamofire/Alamofire
https://github.com/tid-kijyun/Kanna
注意点
httpsのサイトは問題なく動作しますが、httpのサイトだとエラーが出ます。
もし、httpのサイトに接続する場合はinfo.plistをいじる必要があります。
info.plistを開いて、Information Property Listから+で、App Transport Security Settingを追加。同じく+でAllow Arbitary Loadsを追加。最後にValueをYESとします。これでhttpのサイトでもOKです。